![]() | |
Lewis Ngugi |
A question arises here that how much time one should dedicate daily to learn how to code fast and effect? I would say 8 hrs if you are on your own. To reduce the time and effort you need to learn programming, you should benefit from those who have experience and are good at it.
As far as I think, you can boost up your learning process if you learn the basics well. Different authors have a different opinion about what are the basic concepts of computer programming. They are also right. Everyone has own perspective. Here I have listed 5 of the basic concepts of computer programming. I am sure it will save you pretty much time and effort.
I am not saying that you will become an expert in programming after reading this post. I am just helping you to get started, to give you a push. You will get an idea of what are the basics of programming and how these basic concepts work. In order, to get a full understanding of how to code I would recommend you to read 3-5 books of programming fundamentals.
Programming Fundamentals
1. Variables, Constants, and Arrays
Anything that you write in your code is stored in computer memory slots. Each slot has a unique address. To read from and write to a memory location, its address is used. But it is hard to work with numbers like 0x19a823f2... this is how memory addresses look like. Naturally, humans are good at working with names than numbers. That's why a genius (idk who but God bliss him/her) thought that why not to use real world names to deal with computer memory. Variables, constants and Arrays and other similar things are named memory locations. Each offers a different approach to handle memory read/write operations.
You need to remember only 3 things about variables and related terms.
- It is a memory location.
- It has a name.
- It has some data.
Remember, a variable is always a variable. Irrespective of the language that you are using, every variable will have the above-mentioned properties. Once you declare a variable with a name suppose x, a constant or an array the operating system selects one or more memory slots (depends on the type of variable) and attach your given name to these slots. Anywhere you use that x in your code, the mighty Operating System resolves it into the memory address it is attached to. Hope it makes sense.
Where to use?
Use them wherever you have to read/write some data to/from memory again and again in your code.
Constants are the same as variables but you cannot change its value once it is initialized.
An array is just a sequence of memory addresses. It has a name, data type and data. The only difference is that it is just a sequence of memory slots. To use a specific slot in this sequence, indexes are used.
Where to use?
Use constants when you have to write data only once and read many times.An array is just a sequence of memory addresses. It has a name, data type and data. The only difference is that it is just a sequence of memory slots. To use a specific slot in this sequence, indexes are used.
It has also been observed that human remember the things which they see more than the things that they hear. For your convince, a video is included below which further explains the concept.
Where to use?
Use arrays whenever you have a sequence of the same kind of data.
2. Conditional Statements
Conditional statements (the decision is taken based on some condition(s)), also called control statements (control the flow of execution of your code) or if statements (have if keyword in syntax). We will use the term if statement onward.
If statements come to the game when there are multiple choices available and one is to be selected based on a given condition. When there are multiple paths of execution if statements are used to direct the execution flow to one of the paths. It decides whether to execute a block of code or not.
The easiest way to know where to use if statements are to look at your problem statement and identify if word. Any condition following the if word will be your if condition. Real world conditions are depicted in code by using variables and expressions. e.g if a person is at least 18 years old, show him the content (some age-restricted content).
There are different varieties of conditional statements suitable for different scenarios.
Example: if something happens to do something.
Example: if something happens then do something otherwise do something else.
Example: if something happens then do something if something else happens, do something else and keep it going.
Yes, I know, the examples are extremely dumb. Just tried to be more generic. If you have better examples, write in comments. I will include it.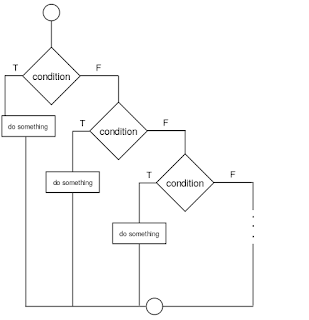
You know what is the function of a printer, don't you? It prints electronic information on a paper. Printing is a sequence of related events. A printer pulls a paper from its tray, prints the queued information on it and pushes it smoothly. Similar to this, a function in programming is a block of code which performs a sequence of related operations. That's it.
If statements come to the game when there are multiple choices available and one is to be selected based on a given condition. When there are multiple paths of execution if statements are used to direct the execution flow to one of the paths. It decides whether to execute a block of code or not.
The easiest way to know where to use if statements are to look at your problem statement and identify if word. Any condition following the if word will be your if condition. Real world conditions are depicted in code by using variables and expressions. e.g if a person is at least 18 years old, show him the content (some age-restricted content).
There are different varieties of conditional statements suitable for different scenarios.
if statement:
Example: if something happens to do something.
if-else statement:
Example: if something happens then do something otherwise do something else.
if-else-if statement:
Example: if something happens then do something if something else happens, do something else and keep it going.
Yes, I know, the examples are extremely dumb. Just tried to be more generic. If you have better examples, write in comments. I will include it.
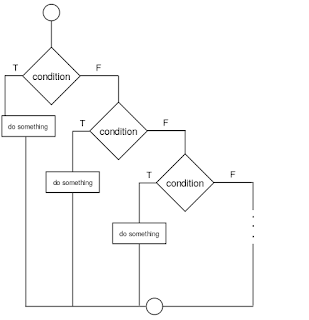
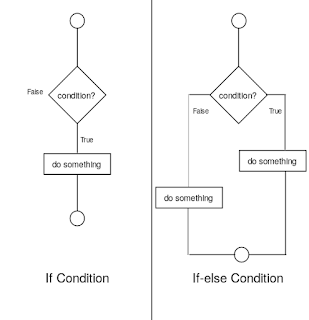
3. Loops
A loop is something which starts from one point, goes around and ends back on starting point. If there is a block of code which you see is consecutively used several times, add it to a loop. Note the word consecutively here. We will refer to it in the next section.
When a block of code is written inside a loop body, it executes again and again. The execution control starts from the first statement of the loop body and progress until it reaches the end. In the end, it returns back to the start of the body. But wait, when will stop executing? Never?
A loop is similar to if statement with an extra feature. The body of a loop is executed again and again based on a conditional expression. If the condition is true, the body is executed, otherwise not. The control returns back to check the condition every time it finishes executing the block of code until the conditional expression evaluates to false. That's why a dynamic conditional statement (not officially called dynamic) is required in loops.
There are 4 widely used variations of loops in popular programming languages like JavaScript, Python, C++, Java. All the variations do the same thing, execute code repeatedly. However, each variation comes with a slight modification suitable for different scenarios.
For Loop
For loop is mostly used as a counter. Like if you want to execute a block of code several times, for loop is the best choice.
Keyword: for
Components:
- initialization
- conditional expression
- condition update statement
- body
While Loop
If you want to run a block of code repeatedly while a condition remains true, go for while loop. You make for loop work like a while loop by ignoring its 1st and 3rd component.
Keyword: while
Components:
- conditional expression
- body
Do-While Loop
The do-while loop comes in handy if you need a block of code at least once whether the condition is true or false. After the first iteration, it works like a while loop. However, in while loop, the condition is evaluated first before its body is executed and in do-while, the condition is evaluated at the end of iteration. Unlike while and for loops, two keywords are used in do-while.
keywords: do, while
Components:
- body
- condition
both components are required.
For-Each Loop
For-each loop is more suitable for situations when you have a list of elements and you want to execute some code for each element of the list. For example, you have a list of some sort of geometrical shapes and want to change the color of each object, use for-each instead of for and while.
keywords: for-each has a syntax similar to for loop in almost all languages. Some languages use each keyword in combination with for and some use : operator.
Components:
- condition
- body
4. Functions / Methods:
Here, we are not talking about the function of a person or things but a function of a block of code. The reason to include the above definition is to present an analogy between functions in the real world and functions in programming.an activity that is natural to or the purpose of a person or thing.
You know what is the function of a printer, don't you? It prints electronic information on a paper. Printing is a sequence of related events. A printer pulls a paper from its tray, prints the queued information on it and pushes it smoothly. Similar to this, a function in programming is a block of code which performs a sequence of related operations. That's it.
Components:
- Function Name
- Parameters
- Return type
- Body
- Function Call
If a function needs input data from another scope then it is given either as a parameter or globally. There are many ways to exchange data between the function body and the rest of the code. Function call necessary for function execution. If there is no call to a function, it will never execute.
When to use functions?
If you have a piece of code for something and you need it more than in one places in your program, enclose it in a function and call it everywhere you need it.
Functions and the upcoming topic deserves more of our time. I will write separate posts for each in detail.5. Class and Objects:
You might have heard that class is a blueprint and an object is an instance of a class. I have read it like a lot time in books and blogs and it was the most confusing statement for me ever. Class and Object are the base concepts of Object Oriented Programming. If you a beginner (most probably you are if you are reading this), it will take you some time and some effort to understand what actually class and objects are and how to better use them. You will get some help from here and a lot more in the coming posts.
What is a Class?
In real-world problems we have several physical and non-physical objects like if you are working on a game, your main character is an object, a weapon is another object, enemies are separate objects. All have some features and functions. Based on their functions, we can categorize these objects. We can put all the similar objects in one category. For example, Weapons are similar to each other while different from humanoid characters.
In OOP, such categories become classes. In the above example, the weapon is one class while the enemy is another class.
In functions, a number of operations which belong to a single event are combined and used again and again with just one function call. Same is the case with classes. Functions which perform operations related to a single category or type of objects are combined into a single class. Obviously, these functions need some data to operate on, so the related data is also encapsulated with these function within the class.
What is an Object?
Can you sleep inside a blueprint of your house? Never. The map/blueprint must first be brought into a physical shape. You have to bring it into existence. It will occupy some space on earth. Yes, don't shout. I know people are trying to live on Mars but it may never happen. But there are crazy inventors are still alive. who knows. Anyhow, same is the case with classes. Once you create a class, it does not occupy any space in computer memory (RAM). So you cannot use it. Sorry.
Unless and until at least one object of that class is created.
The object is used to access methods and data of a class. An object is created almost the same way a variable is created. It has a type (class name) and a name. The same naming conventions are followed to create an object of a class which is followed for functions and variable.
Comments
Post a Comment